Upgrade Guide
Version 2 to 3
Updating to version 3 introduces several breaking changes!
Komments 3 comes with a new data structure for storing comments. Because of this, old comments have to be migrated. The plugin will not delete old comments, to decrease the risk of data loss. But depending on the storage type you chose, it has to write to your page files.
Please:
- Backup your existing data
- Run the migration on a local or test machine before doing this on your live site
Version 3 comes with major changes in all aspects of the plugin, you'll have to adapt your templates and code to the new version.
Storage types
The plugin now is way more flexible in terms of storage types. You can choose between different storage options, such as:
- File storage
- Database storage
File storage will store all comments to your page files as the plugin did before. This works well for sites with a few comments. This storage type does not support all new features, like batch processing. I recommend switching to the database storage type.
Database storage will store all comments in a SQLite database. This is faster and more reliable than file storage. It also supports all new features, like batch processing. If you can switch, I recommend doing so.
SQLite is the new default storage type.
Migration
You have to migrate old comments to the new data structure. There are two ways to do this:
- Use the panel view to migrate the comments (recommended)
- Use the migration Class to migrate comments programmatically
Both options are easy to use. Old comments will not be deleted, you have to clean up after migration. This is important to avoid data loss.
Configure the storage type
Set the storage type in your config.php
. In the case of SQLite, you have to specify the path in which the database is stored. Choose a path where the database file can be written and which may be included in your backup. The content folder might be a good place.
'mauricerenck.komments.storage.type' => 'sqlite',
'mauricerenck.komments.storage.sqlitePath' => '../content/.db/',
If you want to use the file storage type, you need to set the following configuration:
'mauricerenck.komments.storage.type' => 'markdown',
Run the migration
When SQLite is set as a storage type, the plugin will run the database migration when it is initialized the next time. A database file should be created in the specified path.
You also need to migrate old comments. Enable comment migration by setting the following in your config.php
:
'mauricerenck.komments.migrations.comments' => true
Migration in the panel (recommended)
To migrate old comments to the new structure, you can log in to the panel and open the Komments view in the left sidebar.
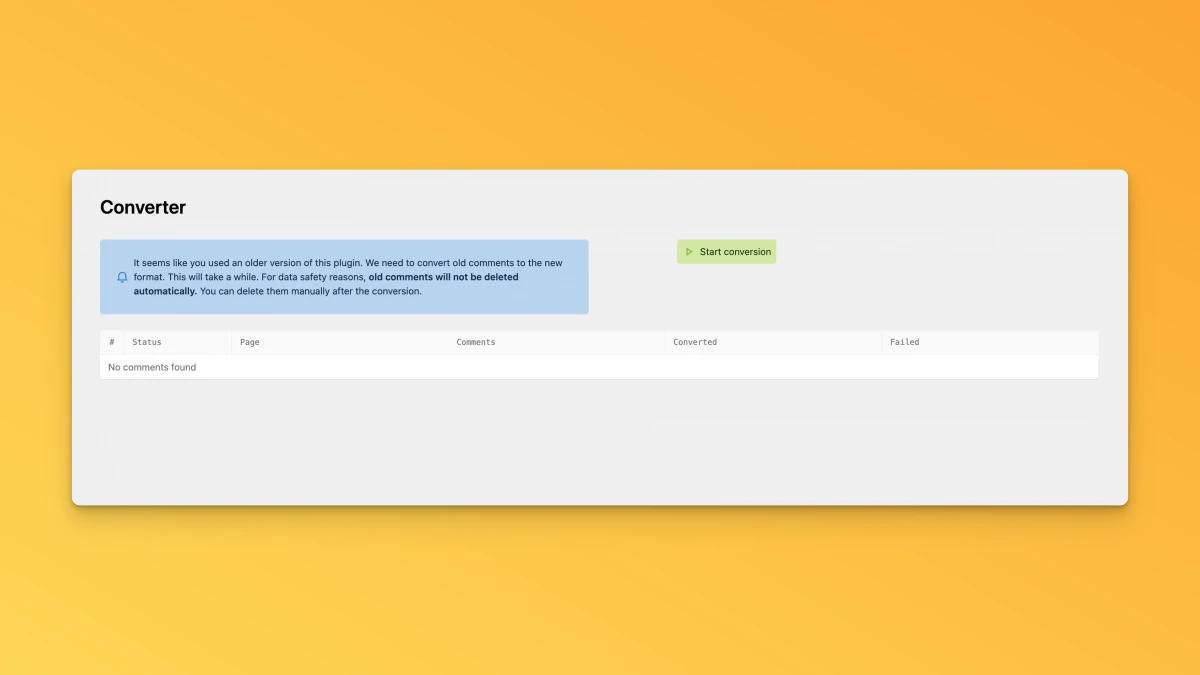
Simply hit the button to start the migration. The plugin will scan all pages for comments, list each page and then starts to migrate each comment. Please do not close the page until the migration is finished.
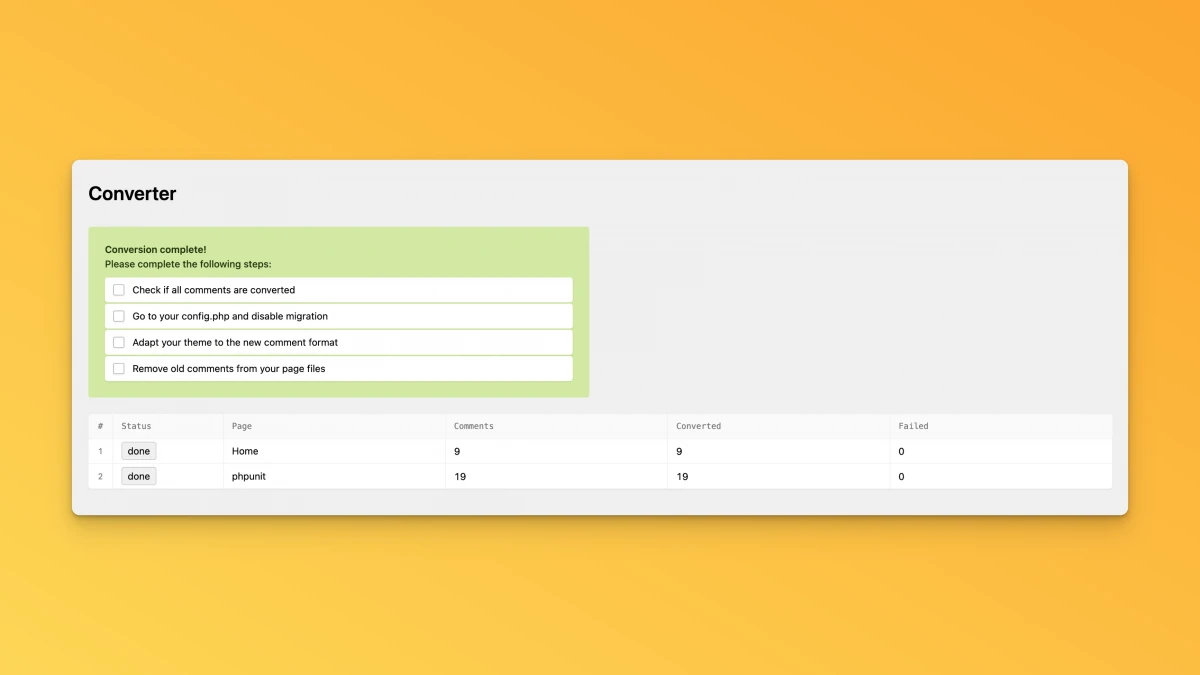
Migration via Migration Class
If you want to run the migration programmatically, you can use the KommentsMigration
class. Here is an example:
<?php
$migration = new mauricerenck\Komments\Migrations();
$migration->convertComments();
?>
This will basically do the same as the panel migration but in one step.
I recommend running the migration via the panel, as you lower the risk of running into timeouts, especially if you have a large number of comments.
After the migration, you can delete the old comments from your page files. You don't need to do this if you don't want to.
When finished, open the config.php
file and set mauricerenck.komments.migrations.comments
to false
. The next time you open the Komments view in the panel, you'll see the regular view with pending comments:
'mauricerenck.komments.migrations.comments' => false
Adapting your templates and snippets
One of the most significant changes is the access to the comments. There are no more arrays of comments and data. Instead, everything is a structure as you know it from Kirby. This way, accessing and filtering comments became way easier. But it also means that you need to adapt your templates and snippets to the new structure if you used custom ones.
Deprecated snippets
snippet name | snippet path | new snippet name | new snippet path |
---|---|---|---|
komments/webmention |
webmentions-splitted.php | DEPRECATED | |
omments/webmention-splitted |
webmentions-splitted.php | DEPRECATED | |
komments/kommentform |
kommentform.php | komments/form | form.php |
komments/type/like |
mention-type-like.php | komments/response/like | response-like.php |
komments/type/reply |
mention-type-reply.php | komments/response/reply | response-reply.php |
komments/type/repost |
mention-type-repost.php | komments/response/repost | response-repost.php |
komments/type/mention |
mention-type-mention.php | komments/response/mention | response-mention.php |
New snippets
snippet | description | filename |
---|---|---|
komments/form |
Shows the comment form | form.php |
komments/response/base |
Basis for each response type customizable by slots | response-base.php |
komments/response/comment |
Shows a single comment | response-comment.php |
komments/response/like |
Shows a single like | response-like.php |
komments/response/mention |
Shows a single mention | response-mention.php |
komments/response/reply |
Shows a single reply | response-reply.php |
komments/response/repost |
Shows a single repost | response-repost.php |
komments/list/comments |
Lists all published comments | list-comments.php |
komments/list/likes |
Lists all published likes | list-likes.php |
komments/list/mentions |
Lists all published mentions | list-mentions.php |
komments/list/replies |
Lists all published replies | list-replies.php |
komments/list/reposts |
Lists all published reposts | list-reposts.php |
Retrieving comments
Comments can now easily be retrieved using a page method instead of the frontend class:
In version 2
$kommentsFrontend = new mauricerenck\Komments\KommentsFrontend();
$commentList = $kommentsFrontend->getCommentList($page);
In version 3
$commentList = $page->comments();
Handling comments and Webmentions
Accessing and handling comments was simplified immensely in version 3. Everything is a Collection or Structure, so you can work with it like you would do with pages or your structures.
Listing responses
In version 2
<?php if ($commentList['replies']->count() > 0) : ?>
<ul class="list-replies">
<?php foreach ($commentList['replies'] as $comment) : ?>
// DISPLAY RESPONSE
<?php endforeach; ?>
</ul>
<?php endif; ?>
In version 3
<?php if ($comments = $commentList->filterBy('type', 'comment')) : ?>
<ul class="list-comments">
<?php foreach ($comments as $comment) ?>
// DISPLAY RESPONSE
<?php endforeach; ?>
</ul>
<?php endif; ?>
Displaying a response
In version 2 every type of response comes with its own snippet. In version 3, every response uses the base-response snippet which has slots defined, individually filled by the response types.
You can access the data of each response as you would do with pages. For example, display the text of a comment:
<?= $comment->content()->kirbytext(); ?>
Here is a list of all available fields.
This should be it. You can follow the other documentations to get more into detail if you want to adjust even more.
Write a comment